Adding Animation using the Cyborg Netcode System
This is optional in most cases, as you could use the built in Unity Animator stuff (check other guide for that). If I add rollback netcode logic to this project then having a predictable animation not relying on floats would help a great deal.
Anyway, the start is similar to the other guide. You need Sprites either single or multi frame first, this appears to be a quick guide on how to do that. We’ll use the Glob assets from the sample project for reference here. You can find the Glob prefab in Assets→Prefabs→Network Entities. The slices for idle down look like this for example:
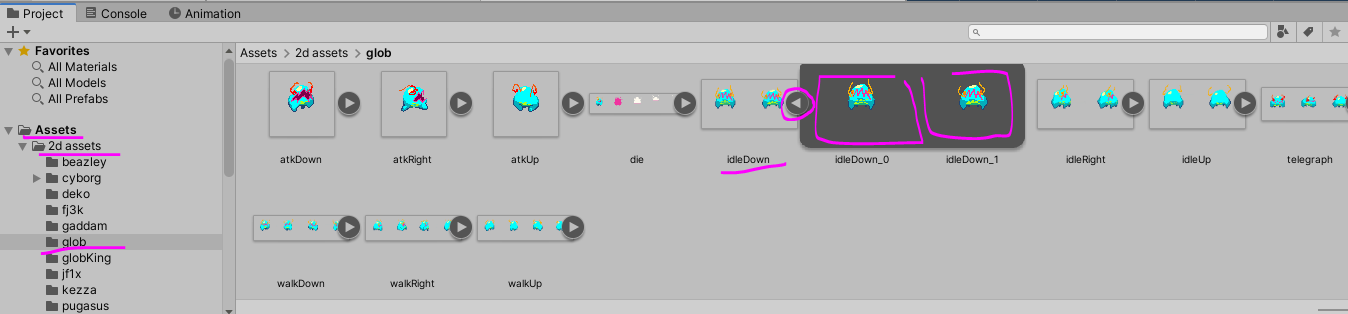
Integrating this with Cyborg Netcode
Add Animation Component onto your NetworkEntity prefab, which is a script found in Assets→Scripts→CyborgNetcode→Components. It needs to reference the Sprite Renderer on your object so it knows how to change the image rendered.
After that its just filling in the data for all of your animation and then dragging your Sprite slices or single Sprites into each frame here. So for on the Glob, it has 14 animations. One of those is IdleDown, we can set if its loopable and then add in the individual frame data. So IdleDown has 2 frames, is loopable and the first frame in that animation is my slice idleDown_0. Duration for frames is measured in Game Loop frames, so if your game loop updates at 60fps, then this first frame will be on screen for 4 of those updates in a second.
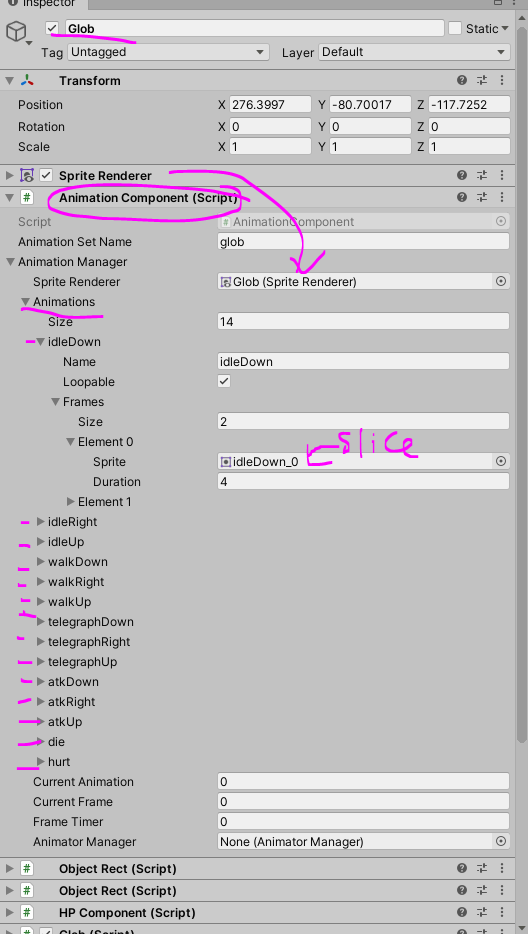
Its not that fancy at this stage, but lets you setup animations in a way that avoid using floats which means they will be deterministic on all machines. Ok, now we want our code to know about this component, so lets rig that up.
The Glob script inherits from the Network Entity class and has the Animation Component reference attribute added to it(not all network entities need animation, so you need to add it to your custom scripts if you need it). So lets make sure its referencing the correct Animation Component
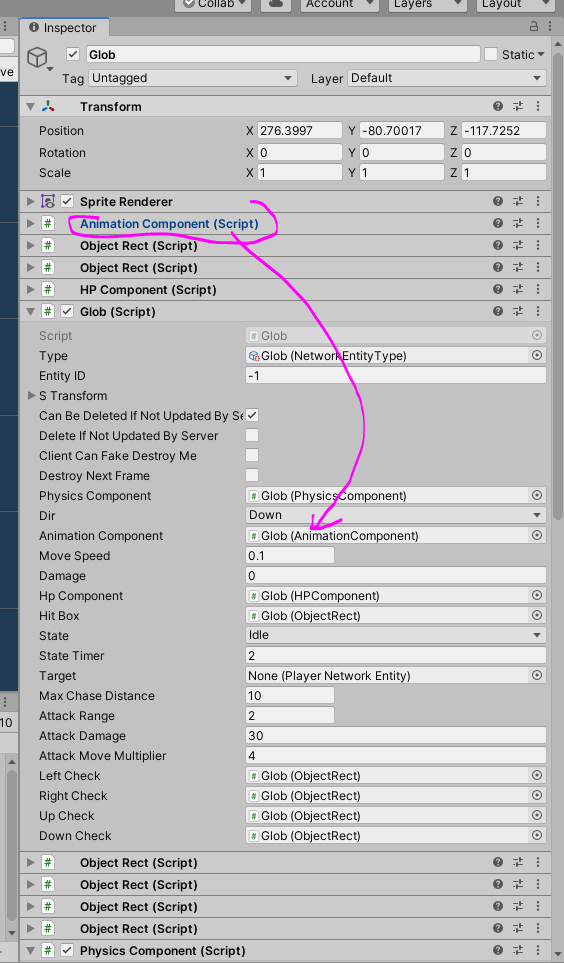
Now to use it in code, we’ll look at the Glob script. When making your own Network Entities, if they have animations, make sure to setup their Animation Component attribute too otherwise you wouldn’t have been able to do the above.
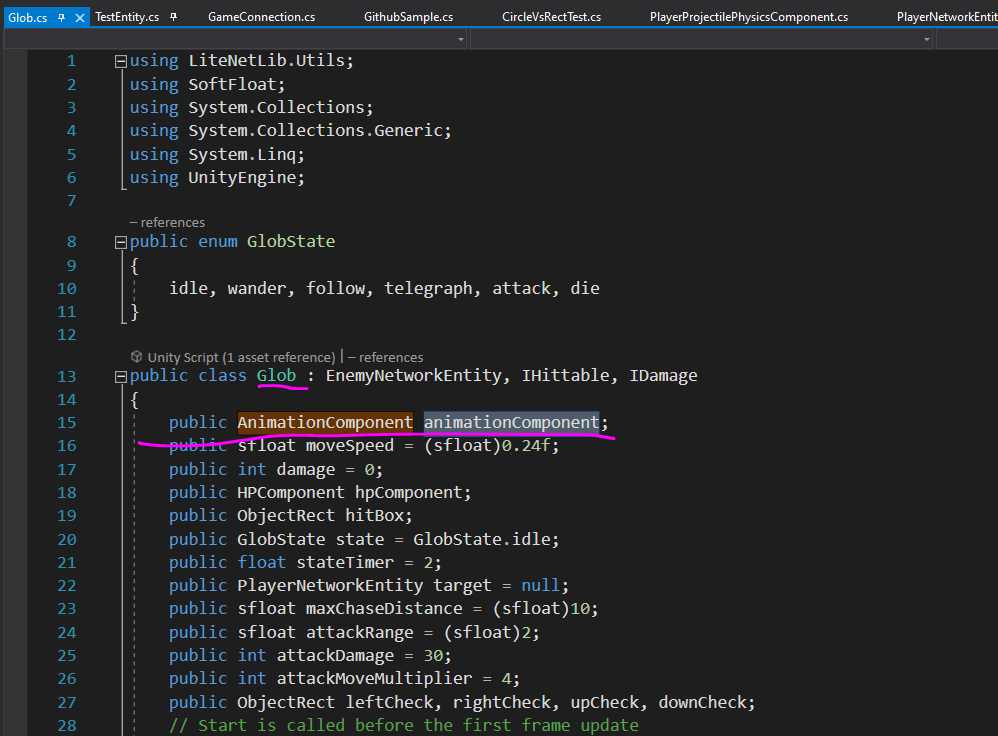
You can check if the current animation loops like this:
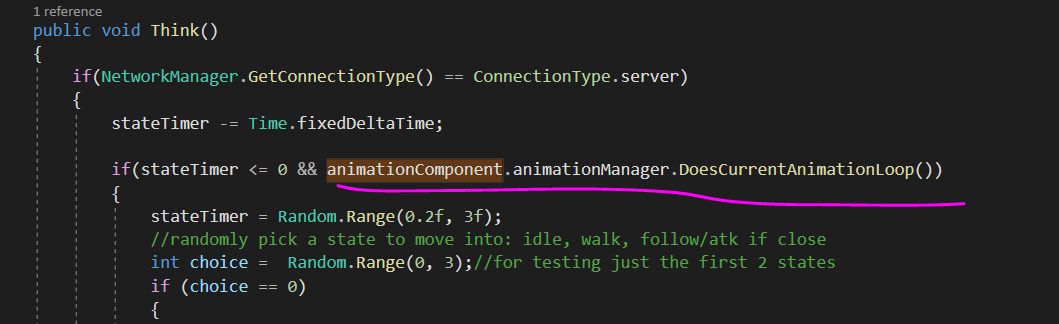
Here is one way to set the current animation on your game object using SetCurrentAnimationIfNotCurrentAnimation but there is also SetCurrentAnimation if you don’t want a conditional outcome.

And there are some helper functions for changing animation. This one takes a direction and figures out which animation by name to pick. Notice Down, Up, Right animation names are put in here, those names were set earlier(like IdleDown shown earlier when in Unity Editor when setting up your animation frames), well Left is not specified because its assuming it will flip the Right image if you need to. There a couple of helper functions, feel free to add your own too.
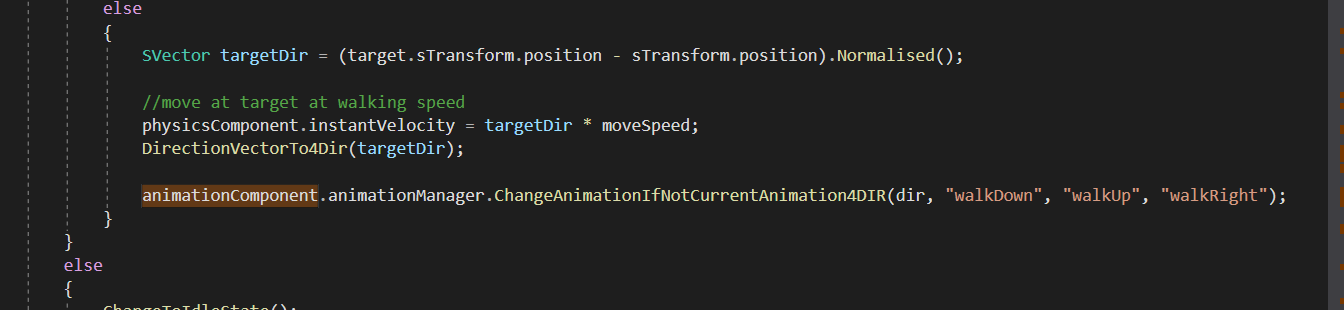
For updating the component in a way we like, here’s an example of how its setup for the Glob
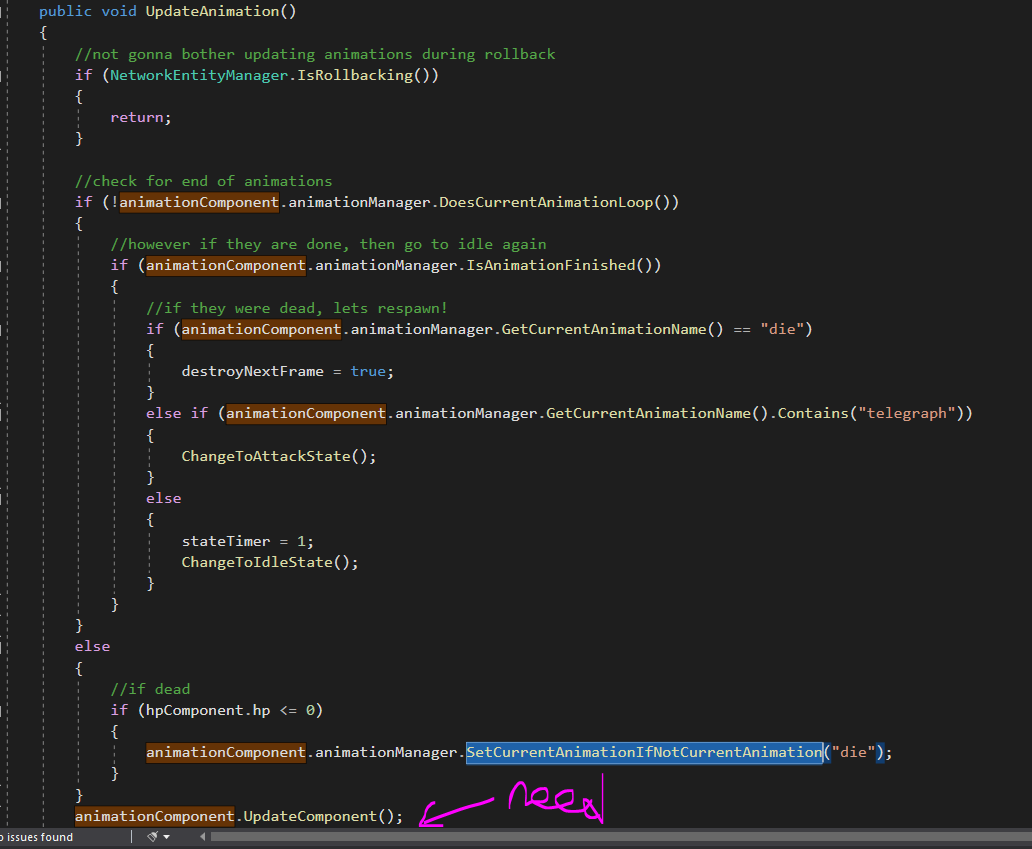
the UpdateComponent method here helps change the sprite on in Unity, so make sure not to skip that.
Updating the component needs to happen in your Network Entities UpdateEntity method to make sure it gets called at the correct time
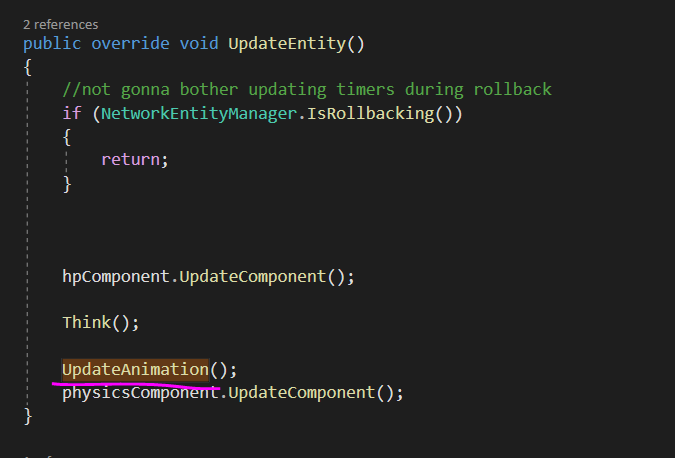
For sending data back and fourth about animation via the network, work with these bits of data
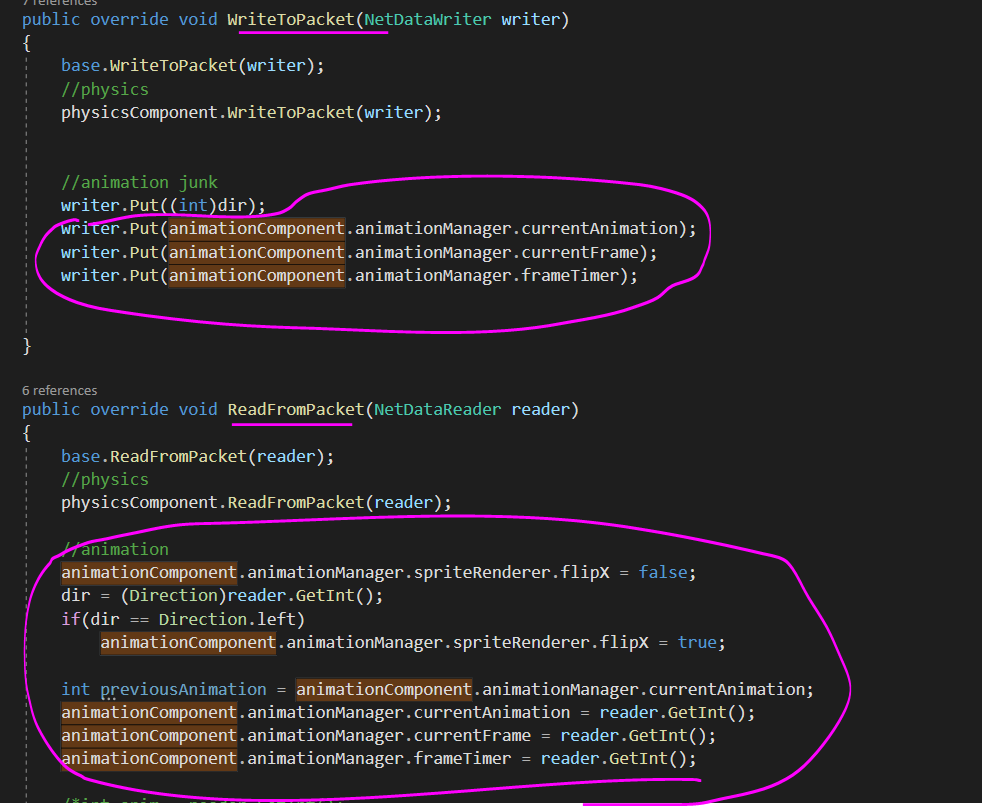
And thats it, go and make some sweet animations on your projects 😀