Adding Animation using the Unity Animation System
I may write up a full tutorial later, but for the moment I’ll reference the sample project. Most of the characters in the sample use my custom animation system, however the GlobKing uses the Unity built in stuff, so that will be our focus for now.
The Sprites
If you haven’t done 2d animation in Unity, then look up Brackeys 2d animation stuff for reference. Basically you need Sprites in your assets folder and if those are in animation strips, you’ll have to split them correctly (here’s a super quick video on sprite splitting). The 2d assets for GlobKing are in 2d Assets→globKing
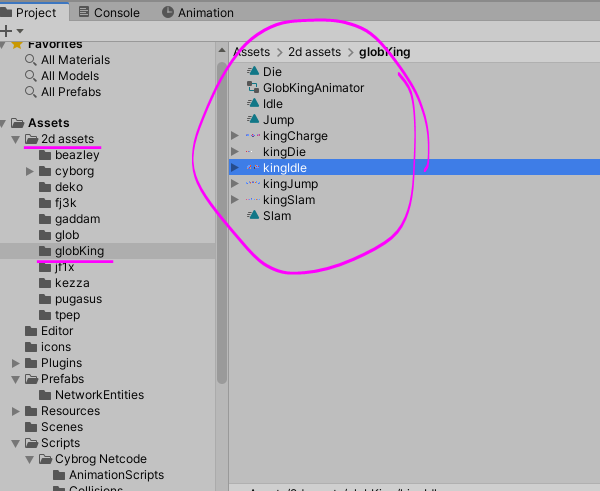
Sprites can be single or multi framed, I’ll use kingIdle for example. Its a 2 frame animation, so its Texture Type is set to Sprite and because its more then 1 frame for this animation, Sprite Mode is set to Multiple. The other settings will depend on your game, but for a quick tip Pixels Per Unit is the default scale of the sprite, the smaller the number the bigger the Sprite appears on screen.
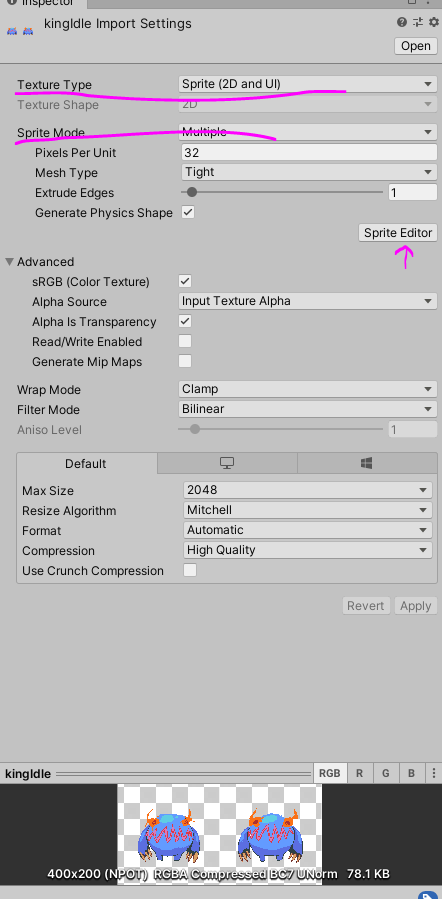
Lets check out the Sprite Editor for this Sprite. In Sprite Editor you can setup origin points of each frame and other useful things. I mostly use it to slice the sprite sheet into multiple frames e.g
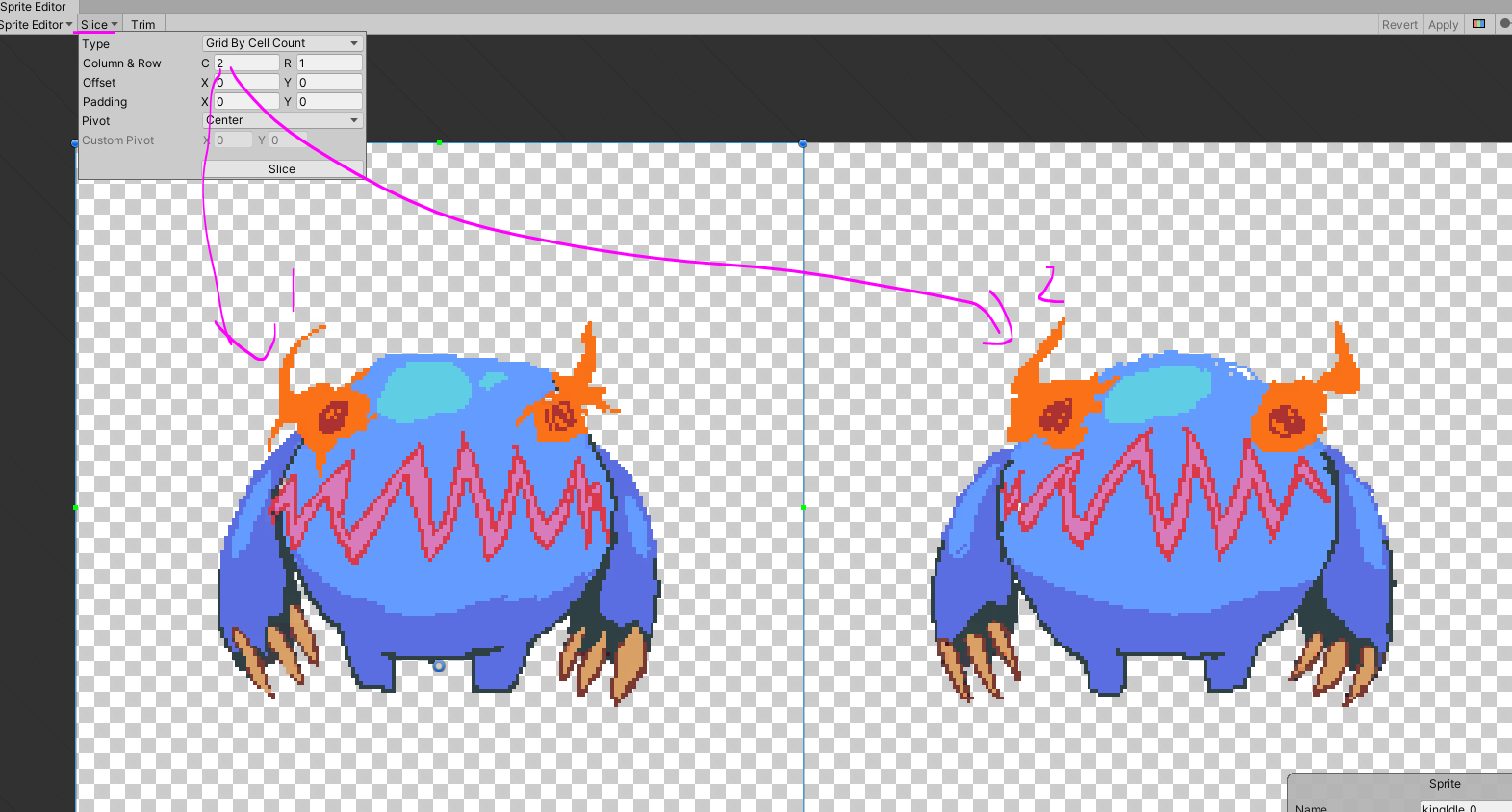
Anyway, look that stuff up if you haven’t done it before. Back in the folder you can expand a sprite if its multiple framed and see the individual textures which you may need to use
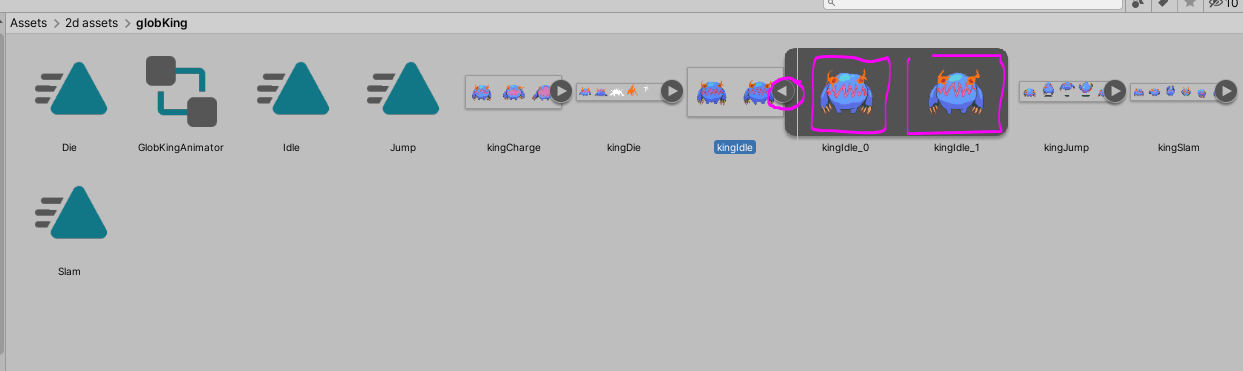
The Animation Manager
An Animation Manager helps animate and transitions animations from one to another on a Unity game object. Its super handy and has a state machine logic to it, again reference some Brackeys videos if you haven’t done this before. You can check out GlobKings Animation Manager stuff by opening up the GlobKing prefab in Assets→Prefabs→NetworkEntities
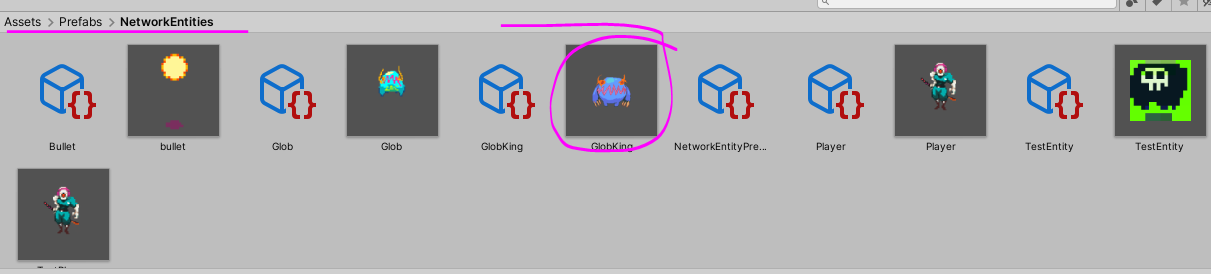
And then make sure you have the Animation and Animator windows open and have a look. You can preview and change any of the animations belonging to the GlobKing in the Animation window, and you can organise the flow of animations for GlobKing in the Animator window.
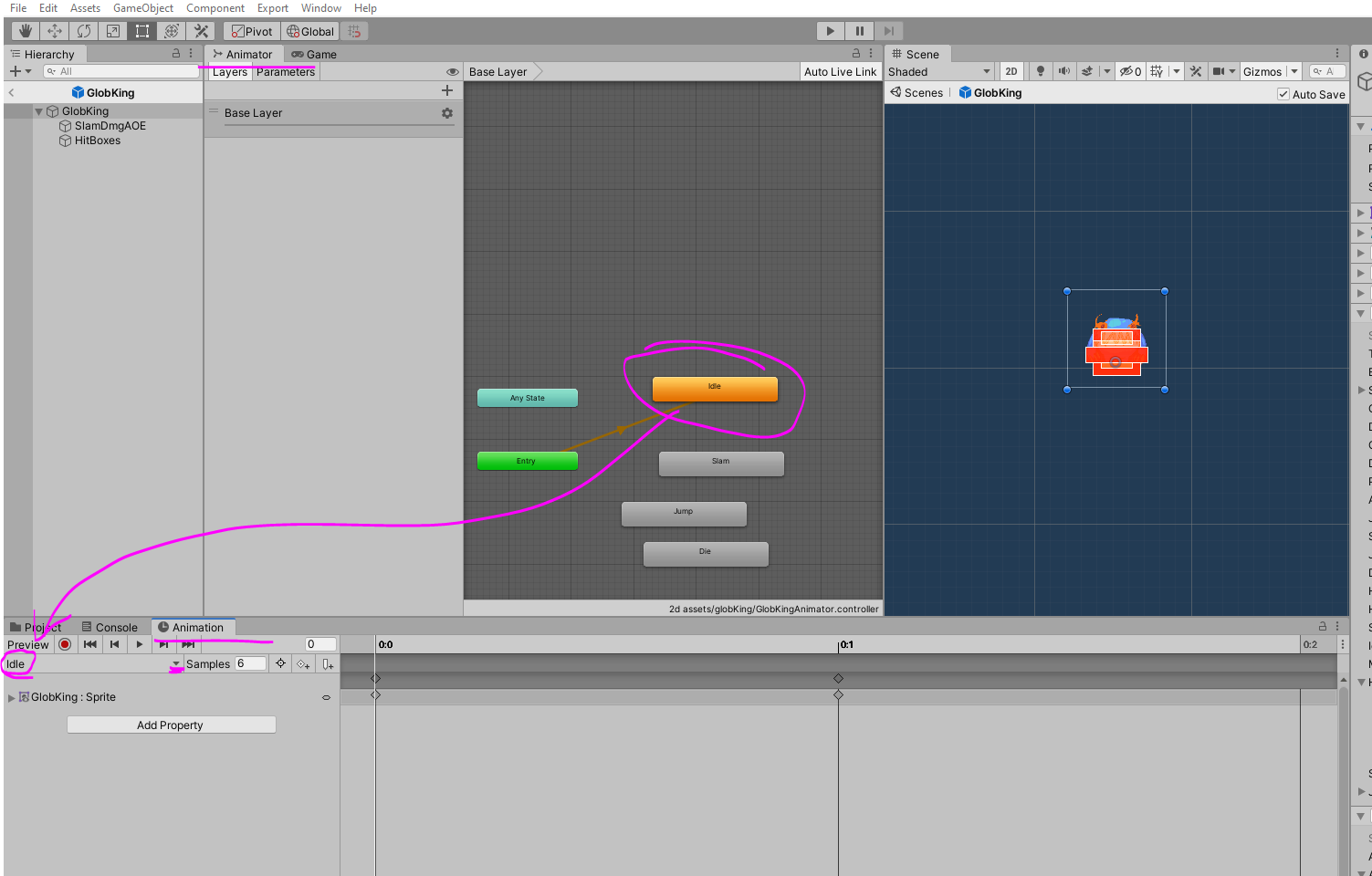
These options are only available because an Animator Controller was setup and assigned to GlobKing via an Animator component
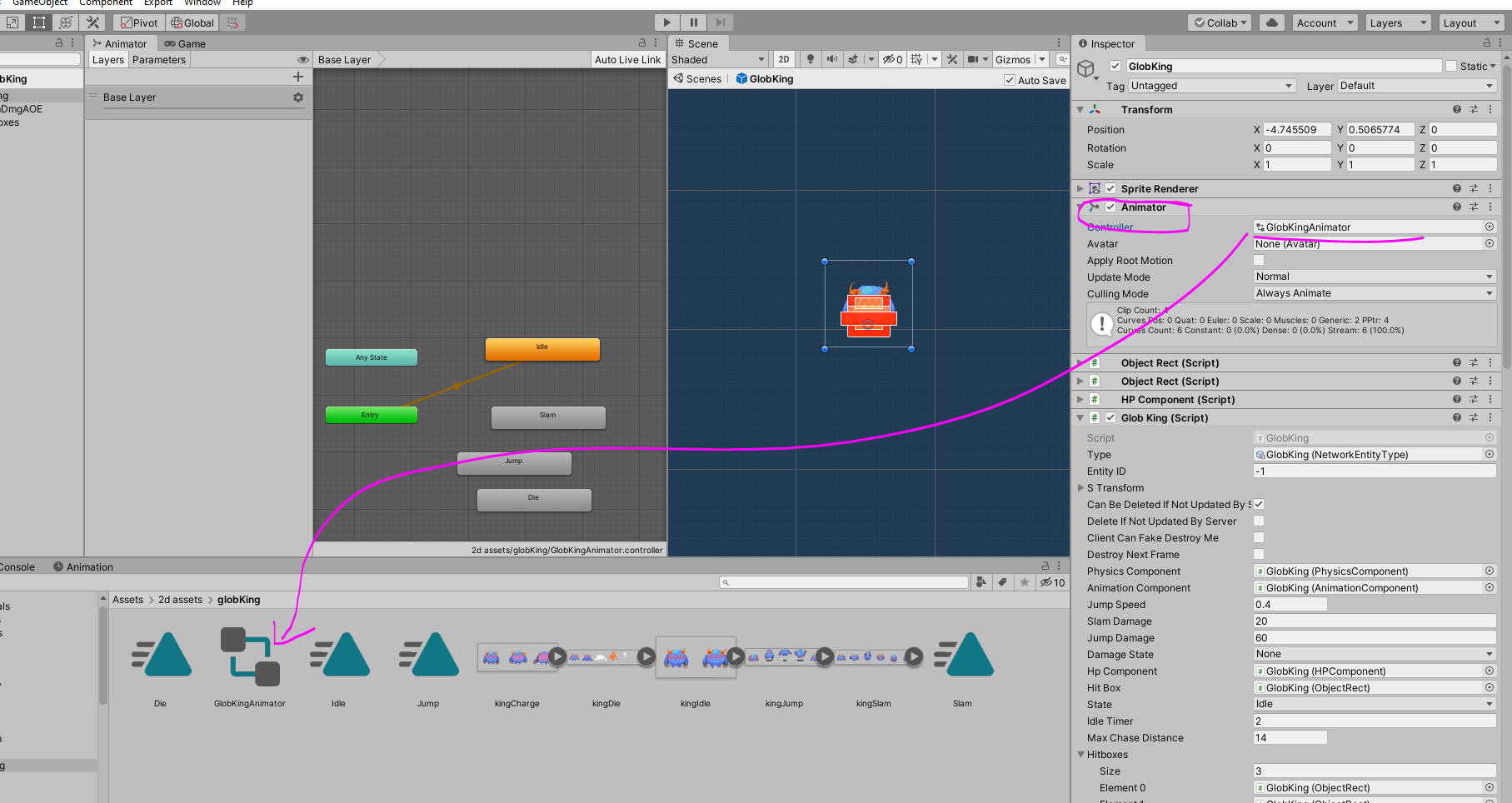
Integrating this with Cyborg Netcode
If you knew how to do the previous stuff, you could have skipped to here. Anyway, add a new component to your entity, the Animation Component which is a script found in Assets→Scripts→CyborgNetcode→Components. Give the animation set a name, choose the Sprite Renderer associated with your current game object/prefab but ignore the animation stuff in there. If we’re using Unity animation stuff, we need a quick little wrapper for help. So also add another component, the Animator Manager which can be found in Assets→Scripts→CyborgNetcode→Animation Scripts and give it reference to the Unity Animator on your game object. Once you’ve done that, get the Animation Component to reference your Animator Manager wrapper component like so.
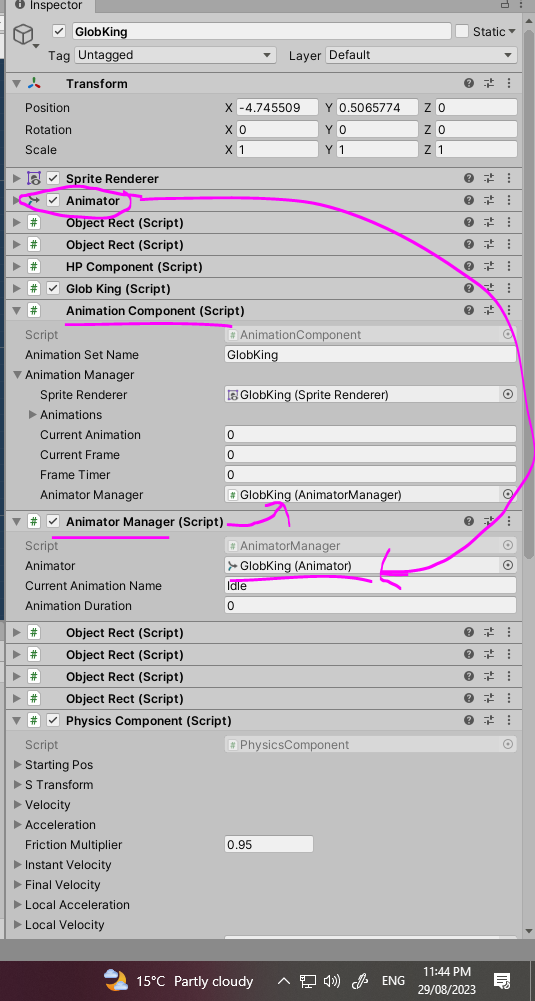
Last thing here is to make sure our Network Entity knows about this Animation Component. This example the GlobKing script extends the NetworkEntity class with added attribute Animation Component(not all network entities need animation, so you need to add it to your custom scripts if you need it), so this is where we setup the animation component:
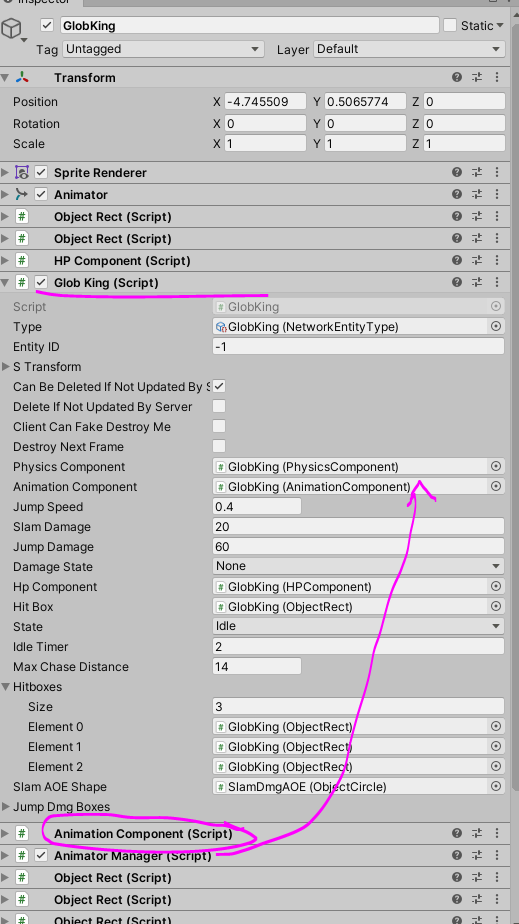
Its a bit to setup, but now you will be able to reference the animations from code when we want animations to change. So lets check out the GlobKing script. If you are making your own NetworkEntity class, make sure it has an AnimationComponent reference like so:
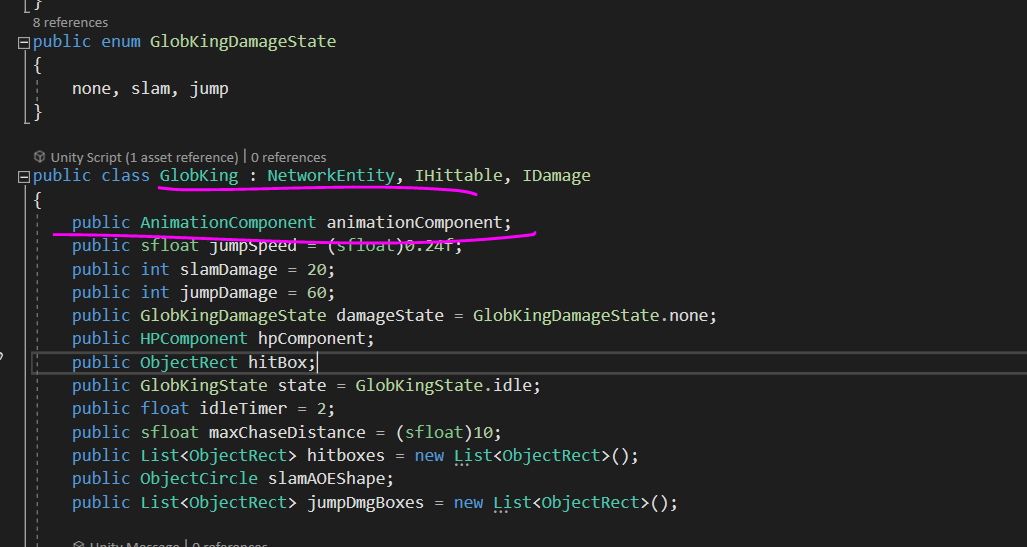
You can check if a certain animation is running or if the current animation loops like so:
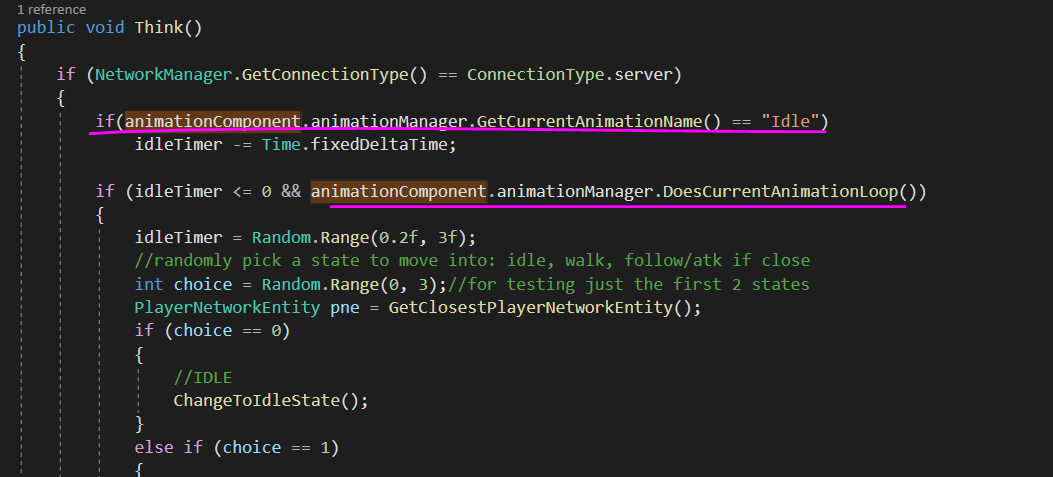
You can change animation by name like this (names match those listed in the animation Manager, the state machine with the animation transitions):
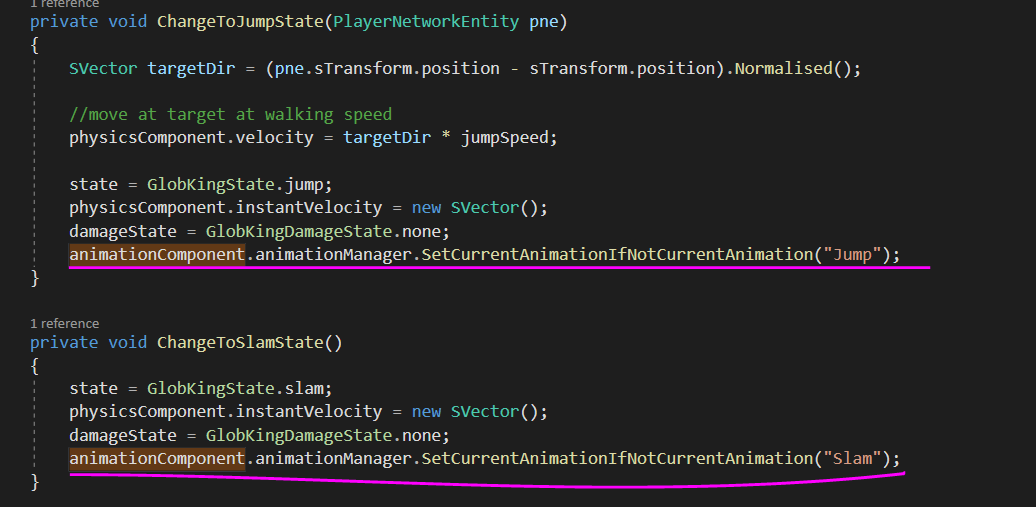
Updating your animations might look like this (though you can change the logic as you like)
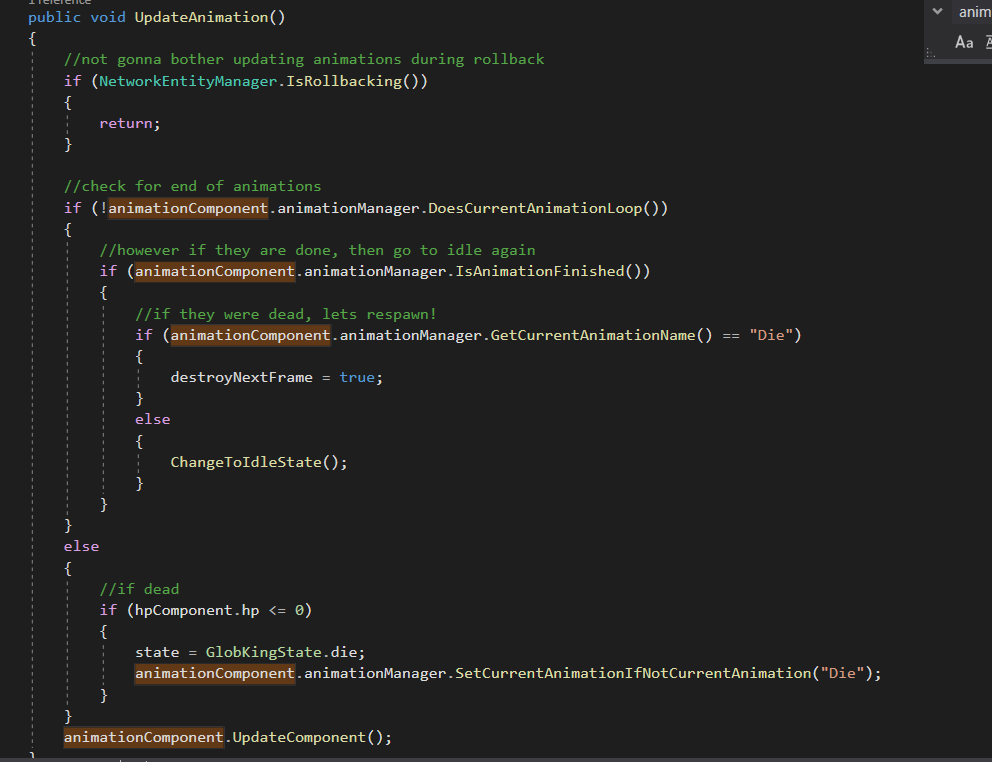
and then just make sure this update function is called in the UpdateEntity method overridden from the NetworkEntity parent class. That ensures it is updated at the correct time in the game loop.
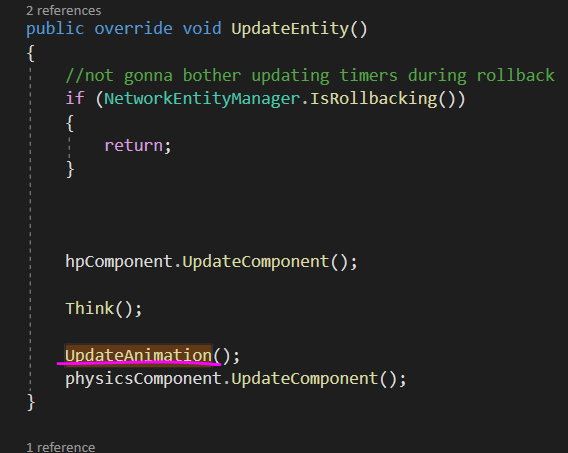
The kind of data to send across the network about animations are these 2 bits of data
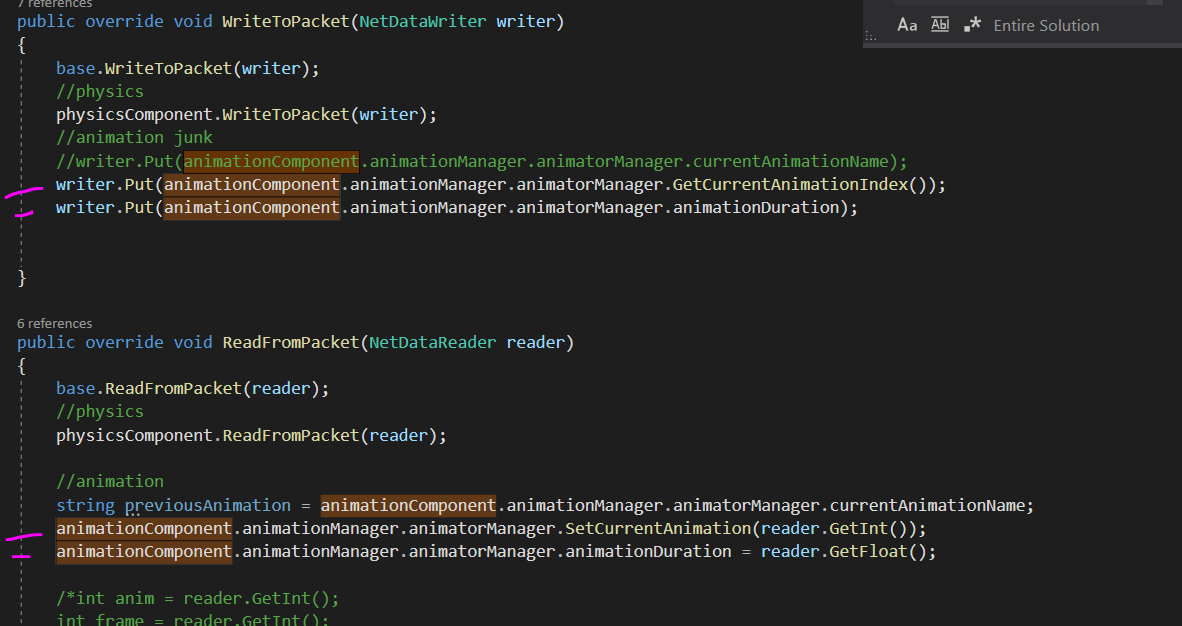
And that’s about it. Prior knowledge of Unity 2d animation was a prerequisite but otherwise not too much work once you hit the coded sections.