Notes on Sounds and VFX
Your game will likely want to have sounds and visual effects, these notes will help guide you on that front.
Sounds
Wherever you want to play sounds with your entities doing actions (e.g player shoots, or shouts something when attacking), then do the code you would normally would for that, but wrap it in a if to make sure the netcode isn’t doing any rollbacks. Rollbacks re-simulate a whole bunch of past frames really quickly, so we really don’t want sound running in there.
Lets see how the player script in the sample deals with sounds. Its called CyborgPlayer(found in Assets→Scripts→CyborgNetcode→Entities) so open that script up in Visual Studio or other code editor. Lets jump to the Slash() method, this is where the player swings their sword and good time for a shwing sound.
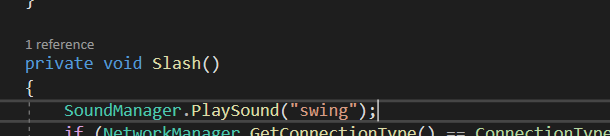
I’m using my own SoundManager class, but really you don’t need to use that. Lets look at that PlaySound method in the SoundManager class
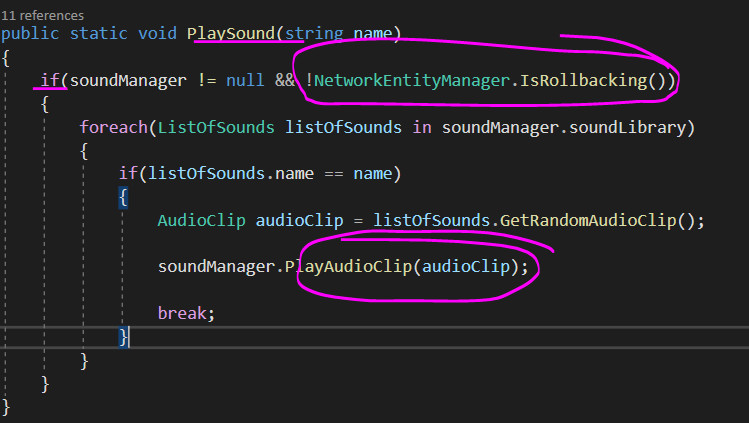
So the most important parts are:
IF the Network Entity Manager is
NOT
rollbacking, THEN play sound.
You can use my sound manager class here or setup your own, just make sure to setup this if statement around it.
If you don’t put this kind of an IF around your sounds, then you may hear multiple sounds get played per frame caused by frames being updated during the rollback phase. don’t want that!
VFX
So with your visual effects (explosions, sparks, particles etc) you need to work out what impact they have on the game from a networked perspective. For each one you want to add to your game ask this question to figure out how to deal with:
Does this VFX interact with any of the Networked Entities in my scene in any meaningful way?
I’ll give some examples to help clarify. So an explosion might get classed as a VFX, but it deals damage or pushback to some entities on screen, where as something like blood spray is usually only a visual thing.
So if they do interact with entities in anyway, then those VFX should be Network Entities and spawned the same way as other networked entities.
If they don’t interact with entities in anyway, then its probably a bit over the top to make them network entities. So make them however you like(particle, MonoBehaviour, whatever), just make sure you don’t create it during the netcodes rollback if its linked to a frame, so use something like this in your entities UpdateEntity function somewhere like so:
if(!NetworkEntityManager.IsRollbacking())
//TODO spawn your effect here
You can also check what animation and frame is on screen to help decide what effects to create within that if block.
OR
Just make sure it can’t be created multiple times in a row due to the rollback
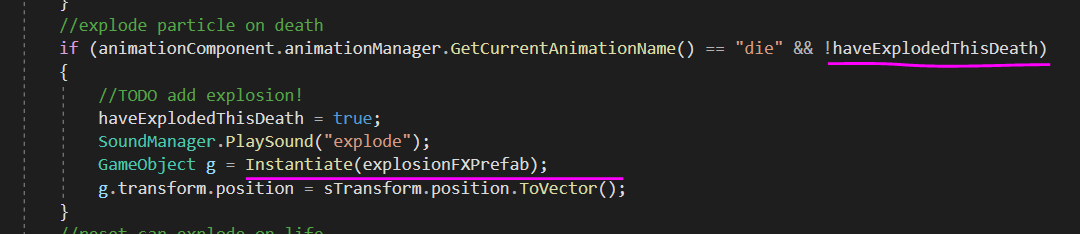
This section is from CyborgPlayer’s Unity Update function and it has a boolean to track whether a soft little non damaging explosion has spawned for the death of this character yet. This boolean gets reset on respawn, so even though its not in the networked update or checking for rollbacks, its pretty safe to call it here. But I wouldn’t use this system for frame specific effects, use the previous approach.
Hope that helps, good luck!