SRect
public class SRect: ICollisionShape
{
public SVector position = new SVector();
public sfloat w = (sfloat)5, h = (sfloat)5;
public Color colour = new Color(0.0f, 1f, 0.0f, 0.1f);
//...
Rectangles are so useful to 2d games. They can represent ground, walls, death pits, punches, parts of the body that take damage and more. If you stripped back the graphics on a lot of 2d games, you would see a bunch of rectangles running around.
A rectangle is made up a position (SVector, so really has x,y,z) and a width and height. Colour is added here too just for visualisation in Unity editor. The position marks the bottom left of the rectangle and the width and height move out to the right and down.
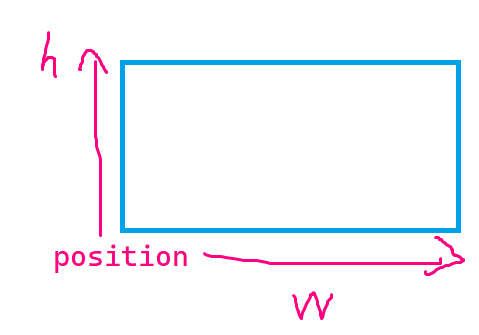
Methods
public SRect()
A basic constructor that sets up a 1x1 green rectangle at position 0,0,0
public SVector GetCentre()
Returns a SVector representing the position of the centre of the rectangle
public SVector GetHalfExtents()
Returns a SVector where the x value is set to half of the width for the rectangle and y is set to half of the height
public SVector GetNegativeHalfExtents()
The same as above but multiplied by -1
public SVector GetTopLeftCorner()
Returns the position of the top left corner of the rectangle
public SVector GetBottomLeftCorner()
Returns the position of the bottom left corner of the rectangle
public SVector GetTopRightCorner()
Returns the position of the top right corner of the rectangle
public SVector GetBottomRightCorner()
Returns the position of the bottom right corner of the rectangle
public List<SLine> GetLines()
Returns a list of 4 lines representing each side of the rectangle
CollisionShapeType ICollisionShape.GetType()
Returns CollisionShapeType.AABB
which means axis aligned bounding box, which is a fancy word for rectangle that’s not rotated