SLine
public class SLine
{
public SVector point1 = new SVector();
public SVector point2 = new SVector();
public Color colour = new Color(0.0f, 1f, 0.0f, 0.5f);
//...
A class representing a simple line between 2 points. Each point is a SVector so although the project is setup for 2d, an SVector has x,y,z components so you can construct lines in 3d if you wish. The colour attribute is purely for visualisation in Unity editor if using a ObjectLine.
Included are some line methods to help with some calculations but you may want to add more.
Methods
public SLine()
Constructor that builds a line between points 0,0,0 and 0,0,0. Not very useful, so make sure to set those line points
public SLine(SVector point1, SVector point2)
Another constructor, this one takes 2 points to describe the line
public sfloat MinX()
Gets the smallest x value from both of the points of the line
public sfloat MaxX()
Gets the largest x value from both of the points of the line
public sfloat MinY()
Gets the smallest y value from both of the points of the line
public sfloat MaxY()
Gets the largest y value from both of the points of the line
public SRect LineToRect()
Returns a rectangle bounding the line which can be helpful in quick collision detections during broadphase. The easiest way to visualise this is with a diagonal line, then it uses the values from the 2 points to be opposite corners of a rectangle. E.g Line pink and the rectangle it generates in blue
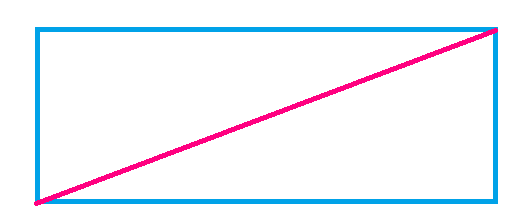